Hi,
Here i am using angular accordion list and codeformer’s angular-drag drop to demonstrate the drag and drop feature.Please have a look at angular-dragdrop and accordion before reading this post.I am using accordion list items as draggable items. I drag the each list item and drop into a square shaped droppable area.Here i am posting a screenshot.
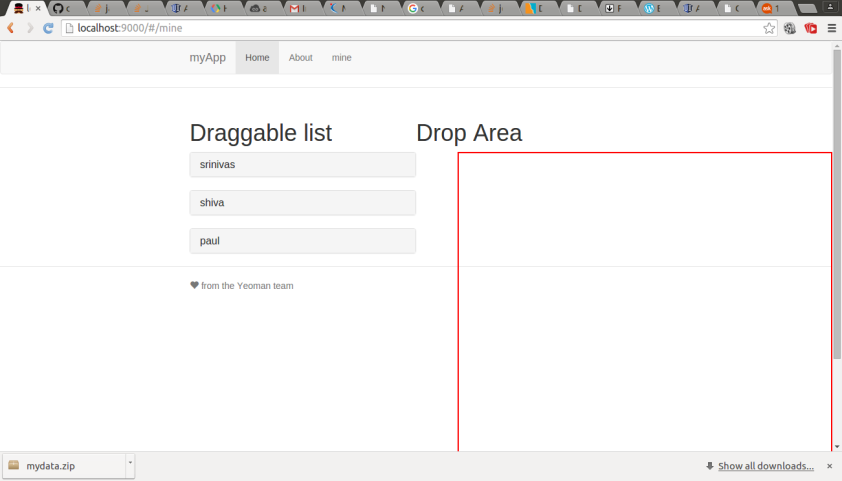
Here is the fiddle i have created for demo. I explain the fiddle’s code in this post.First i go with the draggable list.Here is the code:
<div accordion="" ng-repeat="friend in friends">
<div accordion-group="" class="accord" heading="{{friend.name}}" data-drag="true" data-jqyoui-options="{revert: 'invalid',helper:cloneHandler}" ng-model="friends" jqyoui-draggable="{index: {{$index}},placeholder:'keep',animate:true, onStart:onDragSuccessok(friend, $index)}"></div>
</div>
Here we have two divs one holds the accordion directive and the other have accordion-group directive.They are to generate the accordion list view. In either of these divs we can place our angular-drag drop directive.
1.the data-drag=”true” makes the div element draggable.
2.In jqyoui-options revert:’invalid’ decides Whether the element should revert to its start position when dragging stops..
3.The helper option is to customize the clone.So whats the clone?
When we drag the draggable item, the clone of the draggable item will be extracted and moved.For that we have to explicitly specify the helper:’clone’ option.If we want to customize the clone, we can write our own clone handler like this helper:cloneHandler. The cloneHandler is a function that returns a html template.We can see that in fiddle.
4.In jqyoui-draggable the placeholder option: If true, the place will be occupied even though a dragggable element is moved/dropped somewhere else. If ‘keep’ is supplied, the original item won’t be removed from the draggable.
In progress…..:P